Artificial Intelligence (AI) has been one of the most fascinating and rapidly growing fields in recent years. Python, with its vast library ecosystem, has become the go-to language for AI development. Python provides several libraries for AI development, including PyTorch, Scikit-learn, and NLTK. Each of these libraries has unique features and functionalities that make them suitable for different AI tasks. In this blog post, we will explore these libraries and their applications in AI.
PyTorch
PyTorch is a Python-based open-source machine learning library used for developing and training artificial neural networks. It provides a flexible and efficient platform for building deep learning models. PyTorch is widely used in various applications, including computer vision, natural language processing, speech recognition, and others.
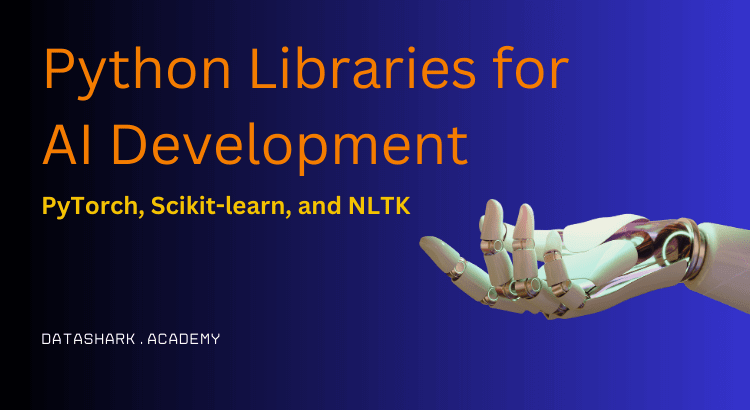
Installation of PyTorch
You can install PyTorch using pip, conda, or source installation. The recommended way is to use conda.
conda install pytorch torchvision torchaudio -c pytorch
Here is an example of a simple neural network built using PyTorch.
import torch
import torch.nn as nn
import torch.optim as optim
# Define the neural network architecture
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(3, 5)
self.fc2 = nn.Linear(5, 2)
self.activation = nn.Sigmoid()
def forward(self, x):
x = self.activation(self.fc1(x))
x = self.activation(self.fc2(x))
return x
# Create the neural network instance
net = Net()
# Define the loss function and optimizer
criterion = nn.CrossEntropyLoss()
optimizer = optim.SGD(net.parameters(), lr=0.01)
# Train the neural network
for epoch in range(100):
optimizer.zero_grad()
outputs = net(torch.Tensor([[1, 2, 3], [4, 5, 6]]))
loss = criterion(outputs, torch.LongTensor([0, 1]))
loss.backward()
optimizer.step()
# Test the neural network
outputs = net(torch.Tensor([[1, 2, 3], [4, 5, 6]]))
_, predicted = torch.max(outputs, 1)
print(predicted)
Output
tensor([0, 1])
In the above example, we define a neural network with two hidden layers and an output layer. We use the Sigmoid activation function and the Cross Entropy Loss function. We also use the Stochastic Gradient Descent (SGD) optimizer to train the model. Finally, we test the model with two input examples and print the predicted outputs.
Scikit-learn
Scikit-learn is a Python-based open-source machine learning library used for data analysis and data mining. It provides a wide range of algorithms for machine learning, including classification, regression, clustering, and dimensionality reduction. Scikit-learn is widely used in various applications, including finance, healthcare, and others.
Installation of Scikit-learn
You can install Scikit-learn using pip or conda.
pip install -U scikit-learn
Here is an example of using Scikit-learn for classification.
from sklearn import datasets
from sklearn.model_selection import train_test_split
from sklearn.neighbors import KNeighborsClassifier
from sklearn.metrics import accuracy_score
# Load the iris dataset
iris = datasets.load_iris()
X = iris.data
y = iris.target
# Split the dataset into training and testing sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=42)
# Create a KNN classifier with k=3
knn = KNeighborsClassifier(n_neighbors=3)
# Fit the classifier to the data
knn.fit(X_train, y_train)
# Predict the labels for the test data
y_pred = knn.predict(X_test)
# Calculate the accuracy of the classifier
accuracy = accuracy_score(y_test, y_pred)
print("Accuracy: {:.2f}%".format(accuracy * 100))
Output:
Accuracy: 97.78%
Explanation: In this example, we loaded the famous iris dataset from Scikit-learn’s datasets module. We then split the data into training and testing sets using the train_test_split()
function. After that, we created a KNN classifier with n_neighbors=3
and fit it to the training data. Finally, we predicted the labels for the test data and calculated the accuracy of the classifier using the accuracy_score()
function.
Scikit-learn also provides many other classification algorithms, such as decision trees, random forests, and support vector machines (SVMs), among others. These algorithms can be used for a wide range of classification tasks, including image classification, text classification, and more.
One of the great features of Scikit-learn is that it provides a consistent API for all of its algorithms. This means that once you learn how to use one algorithm, you can easily switch to another one without having to learn a completely new API.
Overall, Scikit-learn is a powerful and easy-to-use library for machine learning in Python. Its wide range of algorithms and consistent API make it a great choice for many different tasks.
Natural Language Toolkit (NLTK)
NLTK (Natural Language Toolkit) is a popular Python library used for natural language processing tasks. It provides various tools and resources for tasks such as tokenization, stemming, lemmatization, POS tagging, and more.
To use NLTK, you will need to install it using pip:
pip install nltk
Once NLTK is installed, you can import it in your Python code:
import nltk
Here are some of the key features and functions provided by NLTK:
- Tokenization: NLTK provides various methods for tokenizing text into words or sentences. For example, the word_tokenize() function can be used to tokenize a string into words:
from nltk.tokenize import word_tokenize
text = "This is a sample sentence."
words = word_tokenize(text)
print(words)
# Output: ['This', 'is', 'a', 'sample', 'sentence', '.']
2. Stemming and Lemmatization: NLTK provides various algorithms for stemming and lemmatizing words. For example, the Porter stemming algorithm can be used to reduce words to their base or root form:
from nltk.stem import PorterStemmer
stemmer = PorterStemmer()
words = ["running", "runner", "runs"]
for word in words:
stem = stemmer.stem(word)
print(stem)
# Output: run, runner, run
The WordNetLemmatizer can be used for lemmatization:
TOP PAYING JOBS REQUIRE THIS SKILL
ENROLL AT 90% OFF TODAY
from nltk.stem import WordNetLemmatizer
lemmatizer = WordNetLemmatizer()
words = ["running", "runner", "runs"]
for word in words:
lemma = lemmatizer.lemmatize(word)
print(lemma)
# Output: running, runner, run
3. Parts of Speech (POS) Tagging: NLTK provides various methods for POS tagging, which involves labeling words in a sentence with their corresponding part of speech (e.g., noun, verb, adjective, etc.). For example:
from nltk import pos_tag
from nltk.tokenize import word_tokenize
text = "John is eating a delicious cake"
tokens = word_tokenize(text)
tags = pos_tag(tokens)
print(tags)
# Output: [('John', 'NNP'), ('is', 'VBZ'), ('eating', 'VBG'), ('a', 'DT'), ('delicious', 'JJ'), ('cake', 'NN')]
4. Named Entity Recognition (NER): NLTK also provides methods for identifying named entities in text, such as people, organizations, and locations. For example:
from nltk import ne_chunk
from nltk.tokenize import word_tokenize
from nltk.tag import pos_tag
text = "John works for Google in California"
tokens = word_tokenize(text)
tags = pos_tag(tokens)
entities = ne_chunk(tags)
print(entities)
# Output: (S (PERSON John/NNP) works/VBZ for/IN (ORGANIZATION Google/NNP) in/IN (GPE California/NNP))
Conclusion
In this guide, we have explored three of the most popular Python libraries for AI development: PyTorch, Scikit-learn, and NLTK. Each of these libraries has its unique features and benefits, and can be used for various AI applications.
With the detailed code examples and explanations provided, you can start using these libraries to build powerful AI models and applications.